Mastering Django Authentication: Comprehensive Guide with Code Examples
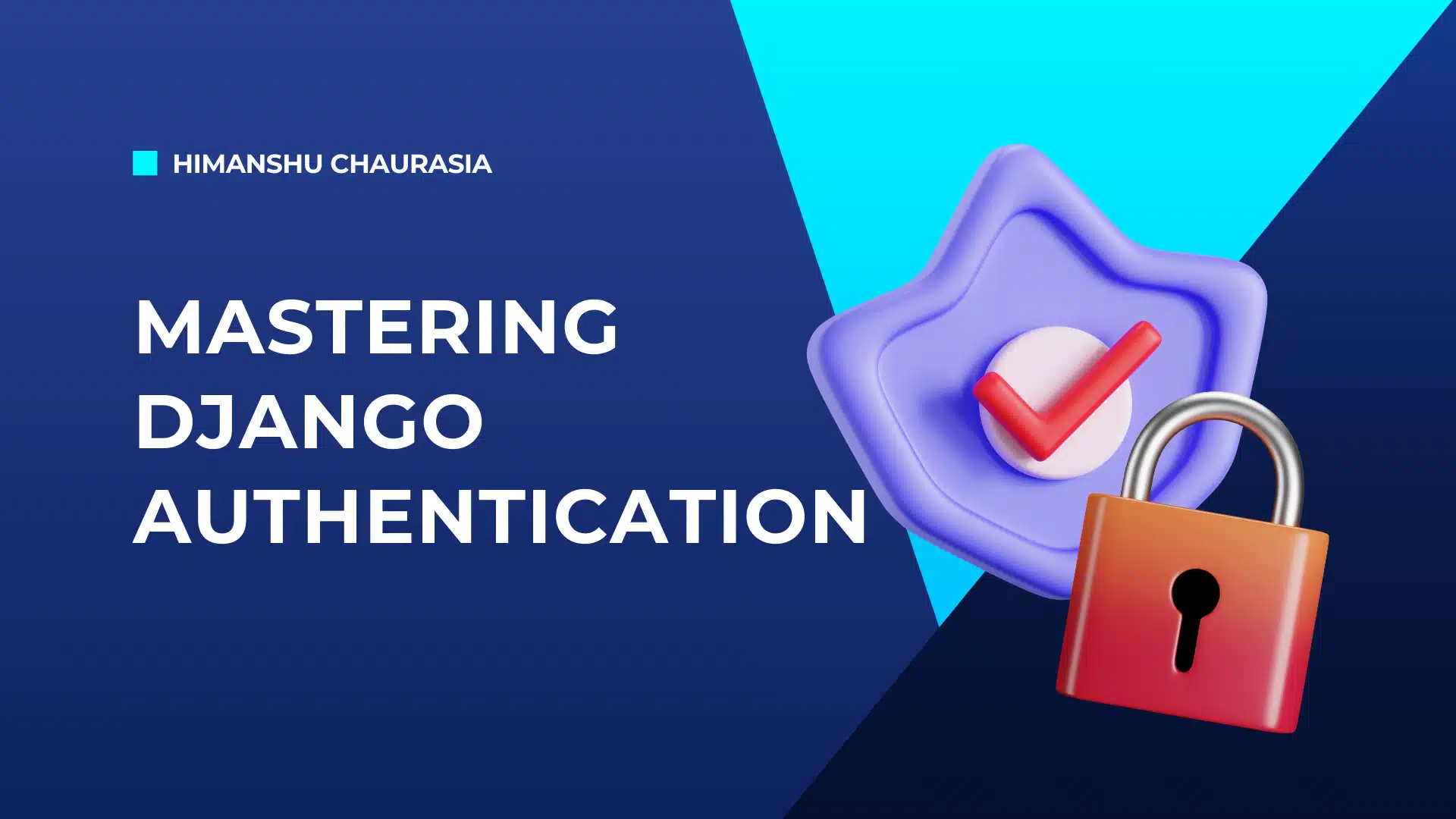
Introduction
Django is a high-level Python web framework known for its simplicity and powerful features. One of its core functionalities is a robust authentication system. This guide aims to provide a comprehensive understanding of Django authentication, with detailed explanations and code examples. Whether you're a beginner or an experienced developer, this tutorial will help you become proficient in Django authentication."
Table of Contents
|
1. Introduction to Django Authentication
Django’s authentication system handles user authentication and authorization, providing built-in views and forms for login, logout, and password management. It includes:
- User management (registration, login, logout)
- Password management (reset, change)
- Authentication backends for custom authentication methods
2. Setting Up Django Project
First, ensure you have Django installed. If not, you can install it using pip:
pip install django
Create a new Django project and app:
django-admin startproject myproject
cd myproject
django-admin startapp accounts
Add the accounts
app to your INSTALLED_APPS
in settings.py
:
# myproject/settings.py
INSTALLED_APPS = [
...
'accounts',
...
]
3. Django Authentication System Overview
Django’s authentication system is built around the User
model, which includes fields like username
, password
, email
, etc. Django also provides authentication views and forms.
4. User Registration
Create a registration form in accounts/forms.py
:
# accounts/forms.py
from django import forms
from django.contrib.auth.models import User
class UserRegistrationForm(forms.ModelForm):
password = forms.CharField(label='Password', widget=forms.PasswordInput)
password2 = forms.CharField(label='Repeat password', widget=forms.PasswordInput)
class Meta:
model = User
fields = ('username', 'first_name', 'email')
def clean_password2(self):
cd = self.cleaned_data
if cd['password'] != cd['password2']:
raise forms.ValidationError('Passwords don’t match.')
return cd['password2']
Create a registration view in accounts/views.py
:
# accounts/views.py
from django.shortcuts import render, redirect
from .forms import UserRegistrationForm
def register(request):
if request.method == 'POST':
form = UserRegistrationForm(request.POST)
if form.is_valid():
new_user = form.save(commit=False)
new_user.set_password(form.cleaned_data['password'])
new_user.save()
return redirect('login')
else:
form = UserRegistrationForm()
return render(request, 'accounts/register.html', {'form': form})
Add the registration URL in accounts/urls.py
:
# accounts/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('register/', views.register, name='register'),
]
Update the project's urls.py
to include accounts
URLs:
# myproject/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accounts.urls')),
]
Create a registration template in accounts/templates/accounts/register.html
:
<!-- accounts/templates/accounts/register.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Register</h2>
<form method="post">
{{ form.as_p }}
{% csrf_token %}
<button type="submit">Register</button>
</form>
{% endblock %}
5. User Login and Logout
Django provides built-in views for login and logout. Update accounts/urls.py
to include these views:
# accounts/urls.py
from django.urls import path
from . import views
from django.contrib.auth import views as auth_views
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
]
Create a login template in accounts/templates/registration/login.html
:
<!-- accounts/templates/registration/login.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Login</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Login</button>
</form>
{% endblock %}
Create a logout template in accounts/templates/registration/logged_out.html
:
<!-- accounts/templates/registration/logged_out.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>You have been logged out</h2>
<a href="{% url 'login' %}">Login again</a>
{% endblock %}
6. Password Management
Django includes built-in views for password reset and change. Update accounts/urls.py
:
# accounts/urls.py
from django.urls import path
from . import views
from django.contrib.auth import views as auth_views
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
path('password_reset/', auth_views.PasswordResetView.as_view(), name='password_reset'),
path('password_reset/done/', auth_views.PasswordResetDoneView.as_view(), name='password_reset_done'),
path('reset/<uidb64>/<token>/', auth_views.PasswordResetConfirmView.as_view(), name='password_reset_confirm'),
path('reset/done/', auth_views.PasswordResetCompleteView.as_view(), name='password_reset_complete'),
path('password_change/', auth_views.PasswordChangeView.as_view(), name='password_change'),
path('password_change/done/', auth_views.PasswordChangeDoneView.as_view(), name='password_change_done'),
]
Create templates for password reset and change views. Example for password reset:
<!-- accounts/templates/registration/password_reset_form.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Reset your password</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Reset password</button>
</form>
{% endblock %}
7. User Profile Management
Create a view to handle user profiles:
# accounts/views.py
from django.contrib.auth.decorators import login_required
@login_required
def profile(request):
return render(request, 'accounts/profile.html')
Add a URL for the profile view in accounts/urls.py
:
# accounts/urls.py
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
path('profile/', views.profile, name='profile'),
path('password_reset/', auth_views.PasswordResetView.as_view(), name='password_reset'),
path('password_reset/done/', auth_views.PasswordResetDoneView.as_view(), name='password_reset_done'),
path('reset/<uidb64>/<token>/', auth_views.PasswordResetConfirmView.as_view(), name='password_reset_confirm'),
path('reset/done/', auth_views.PasswordResetCompleteView.as_view(), name='password_reset_complete'),
path('password_change/', auth_views.PasswordChangeView.as_view(), name='password_change'),
path('password_change/done/', auth_views.PasswordChangeDoneView.as_view(), name='password_change_done'),
]
Create a profile template in accounts/templates/accounts/profile.html
:
<!-- accounts/templates/accounts/profile.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Profile</h2>
<p>Welcome, {{ user.username }}!</p>
{% endblock %}
8. Custom Authentication Backends
Django allows the creation of custom authentication backends. Create a custom backend in accounts/backends.py
:
# accounts/backends.py
from django.contrib.auth.backends import BaseBackend
from django.contrib.auth.models import User
class EmailBackend(BaseBackend):
def authenticate(self, request, username=None, password=None, **kwargs):
try:
user = User.objects.get(email=username)
if user.check_password(password):
return user
except User.DoesNotExist:
return None
def get_user(self, user_id):
try:
return User.objects.get(pk=user_id)
except User.DoesNotExist:
return None
Add the custom backend to AUTHENTICATION_BACKENDS
in settings.py
:
# myproject/settings.py
AUTHENTICATION_BACKENDS = [
'accounts.backends.EmailBackend',
'django.contrib.auth.backends.ModelBackend',
]
Django's authentication system is powerful and flexible, providing essential features for secure user management. By following this guide, you now have a comprehensive understanding of how to implement and customize Django authentication.
Mastering Django authentication is crucial for building secure and user-friendly applications. Keep exploring and enhancing your skills to create robust Django applications.
FAQs
Que 1. How do I customize the user model in Django?
Ans. Use Django’s AbstractUser or AbstractBaseUser to create a custom user model.
Que 2. How can I add social authentication (Google, Facebook) to my Django app?
Ans. Use third-party packages like django-allauth or social-auth-app-django
Que 3. How do I handle email verification in Django?
Ans. Use Django’s built-in email system to send verification emails and create views to handle the verification process.
About Author
Latest Blogs
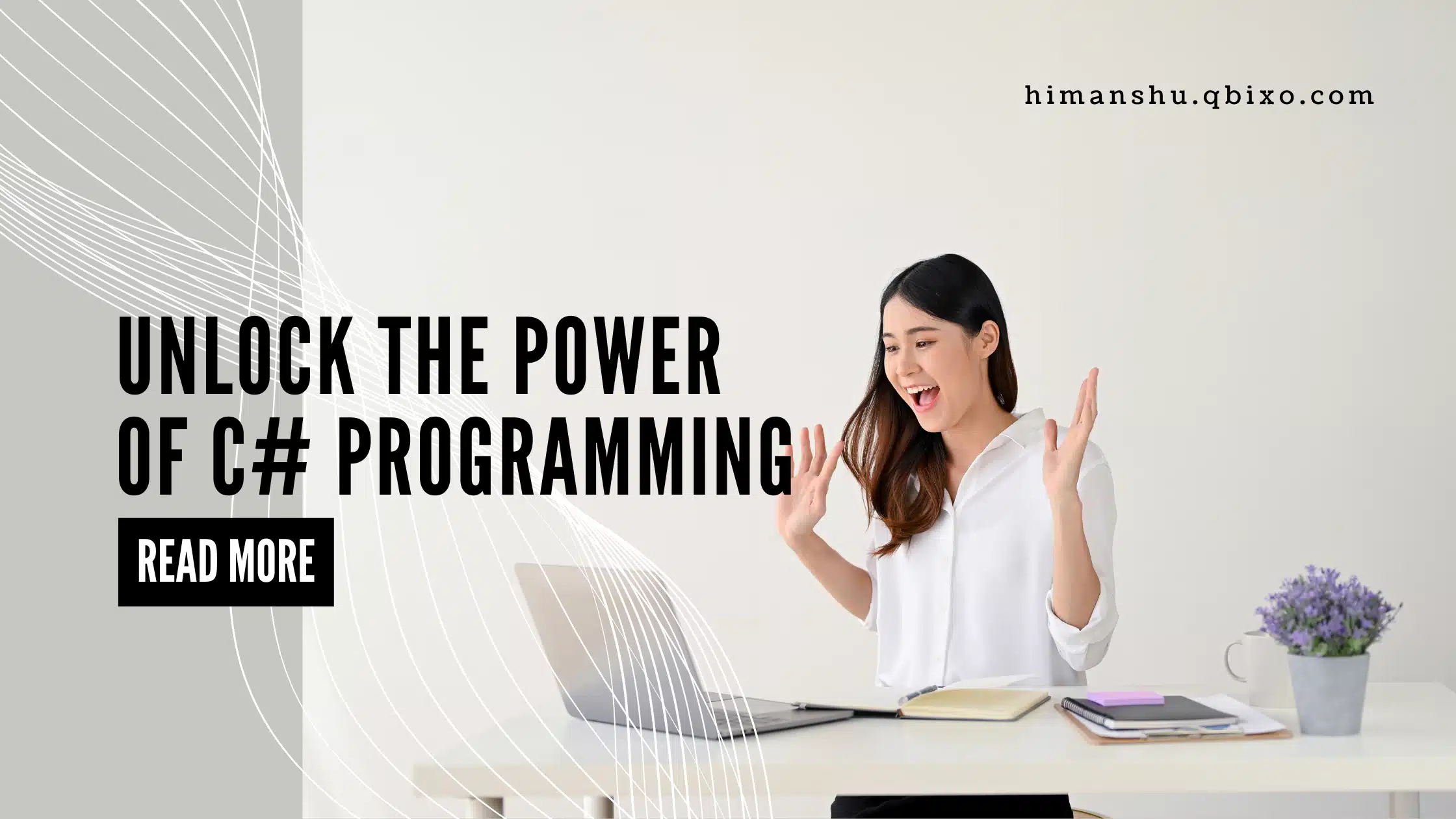
Mastering C#: Your Ultimate Guide to Learning C# Programming
Introduction to C#C# (pronounced "C sharp") is a versatile and powerful programming language developed by Microsoft. Launched in the early 2000s, it is primarily used for building Windows applications, web services, and games. With its clean syntax and object-oriented principles, C# has become one of the most popular programming languages worldwide.Why Learn C#?Versatility: C# is used in various domains, from desktop applications to cloud-based services.Strong Community: With a robust community …
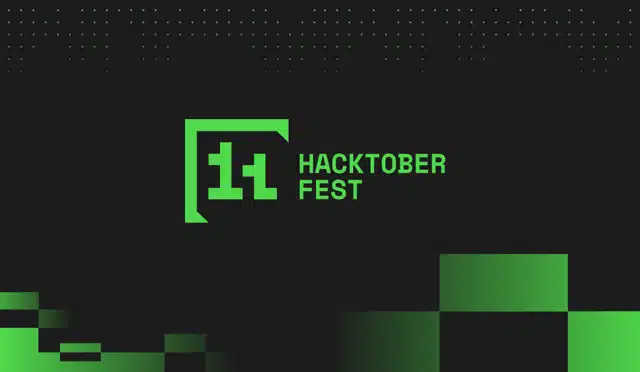
A Complete Guide to Hacktoberfest 2024: How to Register, Contribute, and Make the Most of It
Hacktoberfest is back for 2024! This annual event encourages developers worldwide to contribute to open-source projects. Whether you're a seasoned open-source contributor or a newcomer, this guide will walk you through the process of getting started, making contributions, and maximizing your participation in Hacktoberfest 2024. What is Hacktoberfest?Hacktoberfest is an event held every October to celebrate and promote open-source software. DigitalOcean organizes it in partnership with other tech companies and open-source …
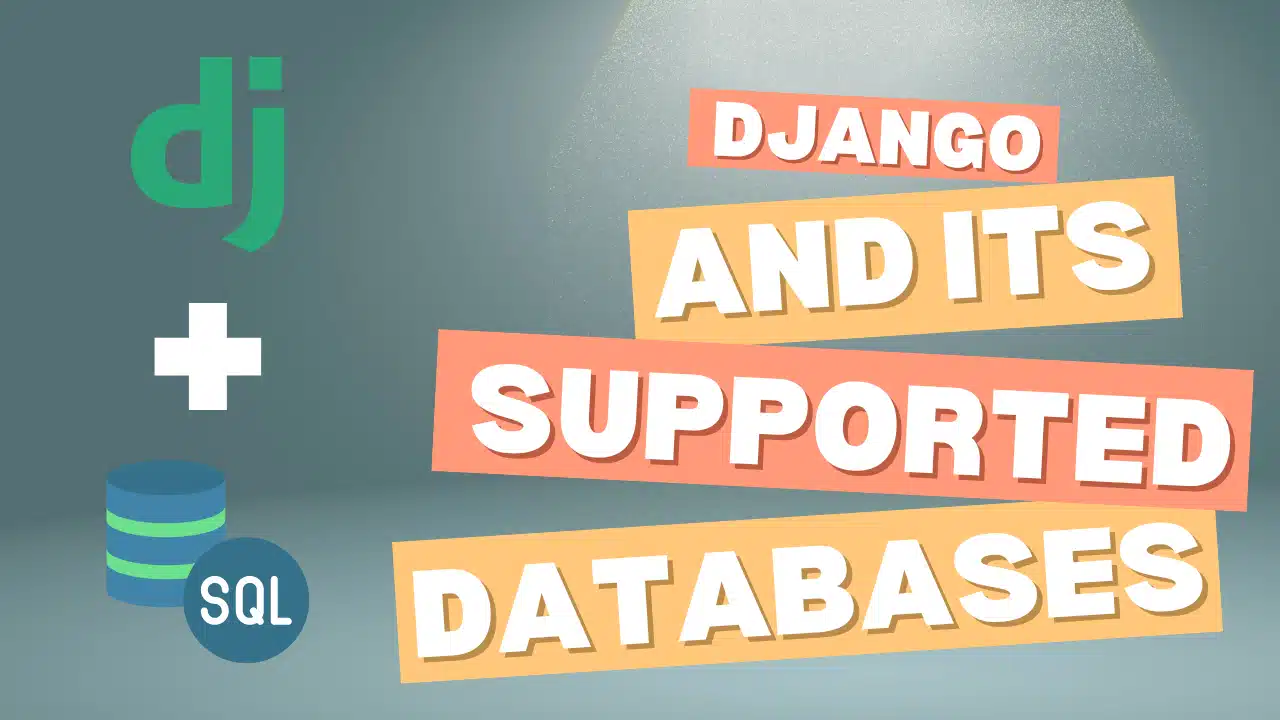
Django and Its Supported Databases: A Comprehensive Guide
Django, a powerful web framework written in Python, offers seamless integration with various databases. Choosing the right database depends on your project needs. This guide will explore all available databases compatible with Django, how to connect them, incompatible databases, and frequently asked interview questions related to Django database integration.Supported Databases in DjangoPostgreSQLMySQLMariaDBSQLiteOraclePostgreSQLPostgreSQL is a popular open-source relational database that is fully supported by Django. It's known for advanced features like …
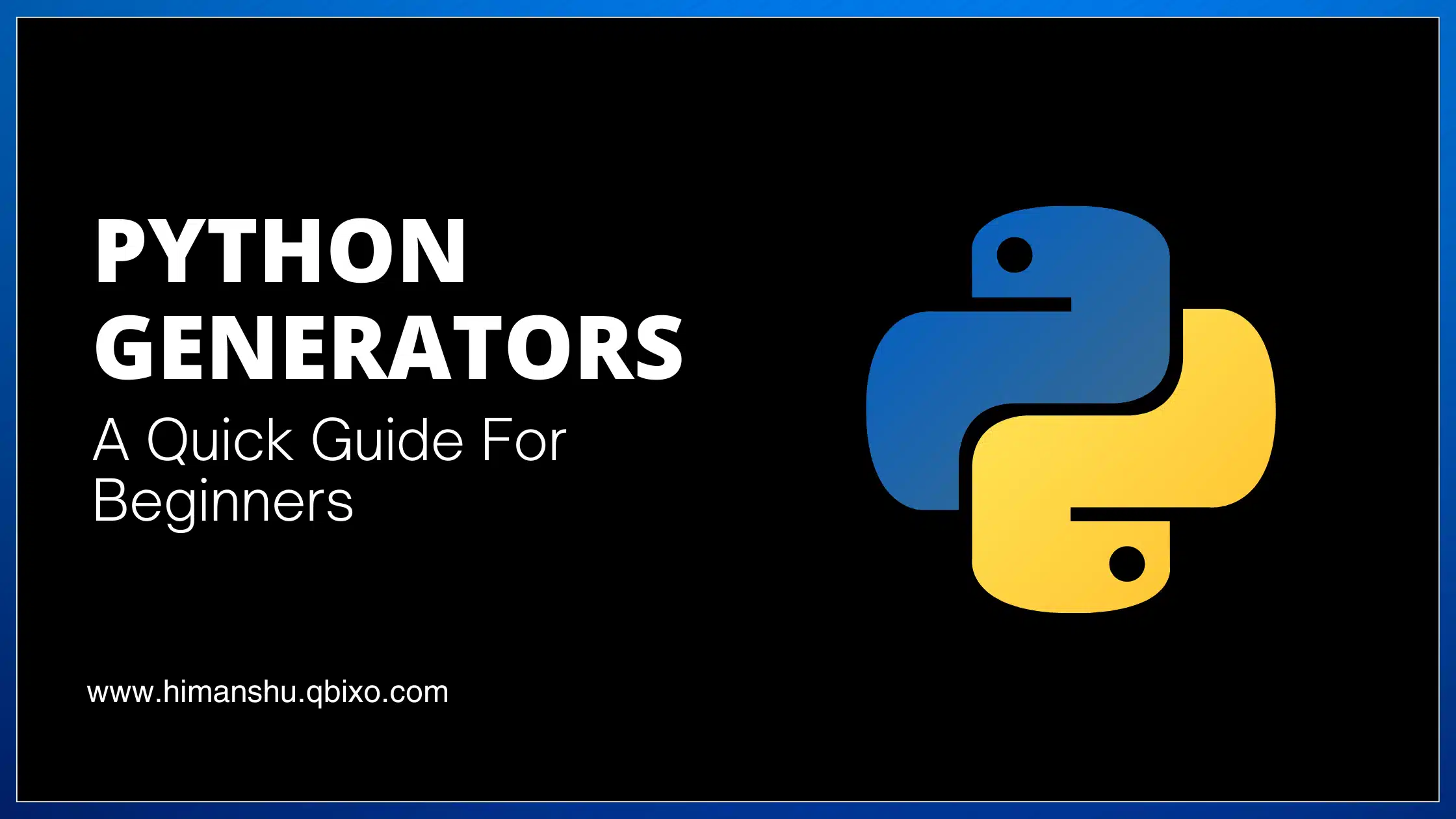
Python Generators: A Comprehensive Guide with Examples, Use Cases, and Interview Questions
IntroductionIn Python, generators provide a powerful tool for managing large datasets and enhancing performance through lazy evaluation. If you’re aiming to optimize memory usage or handle streams of data efficiently, understanding Python generators is crucial. This blog will cover what Python generators are, how they work, their advantages, scenarios where they shine, and some common interview questions. Whether you're a seasoned developer or new to Python, this guide will help …
Social Media
Tags
#Django
#DjangoAuthentication
#WebDevelopment
#Authentication