Python Generators: A Comprehensive Guide with Examples, Use Cases, and Interview Questions
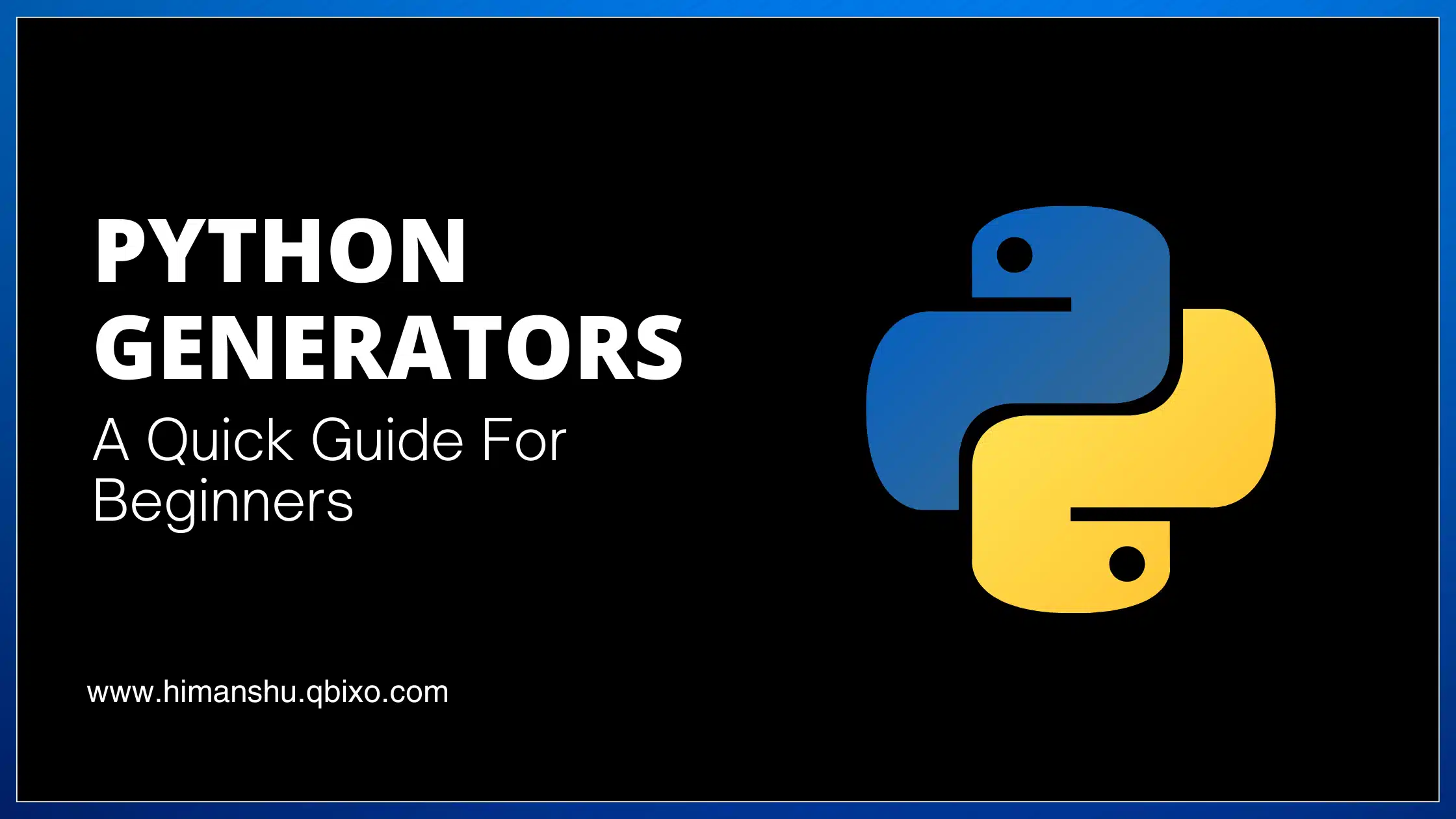
Introduction
In Python, generators provide a powerful tool for managing large datasets and enhancing performance through lazy evaluation. If you’re aiming to optimize memory usage or handle streams of data efficiently, understanding Python generators is crucial. This blog will cover what Python generators are, how they work, their advantages, scenarios where they shine, and some common interview questions. Whether you're a seasoned developer or new to Python, this guide will help you master generators.
What Are Python Generators?
Python generators are a special class of functions that return an iterable sequence of values, one at a time, without storing them all in memory. Unlike regular functions that use return
, generators use the yield
keyword to produce a series of results over time. This makes them an excellent choice for iterating over large datasets or streams of data.
An example of a Simple Generator
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
for value in gen:
print(value)
Output:
1
2
3
In the example above, the simple_generator()
the function yields values one by one. Each time the generator is called, it resumes where it left off, producing the next value until it’s exhausted.
Why Use Python Generators?
Generators offer several advantages that make them a preferred choice in various scenarios:
- Memory Efficiency: Generators don’t store the entire sequence in memory; they generate values on the fly. This is especially useful when dealing with large datasets or infinite sequences.
- Improved Performance: Because they yield values one at a time, generators are faster and more efficient for large loops or data streams, as they avoid the overhead of storing all values in memory.
- Lazy Evaluation: Generators evaluate values only when needed, which can lead to significant performance gains, particularly in pipelines or real-time data processing.
- Cleaner Code: Generators allow you to write more concise and readable code, especially when dealing with complex data processing tasks.
Scenarios Where Generators Shine
Generators are particularly useful in the following scenarios:
Processing Large Files: When working with large text or binary files, you can use generators to process the file line by line or chunk by chunk without loading the entire file into memory.
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()Infinite Sequences: Generators are ideal for creating infinite sequences, such as generating an endless series of numbers.
def infinite_numbers(start=0):
while True:
yield start
start += 1
gen = infinite_numbers()Data Streaming: Generators can be used to stream data from external sources (like APIs) in real time, handling data as it arrives without blocking the program.
import requests
def stream_data(url):
response = requests.get(url, stream=True)
for chunk in response.iter_content(chunk_size=1024):
yield chunkEfficient Pipelines: Generators can be chained together to form pipelines, where each generator processes data and passes it to the next. This is particularly useful in data processing tasks.
def pipeline_example(data):
for item in data:
yield item * 2
Conclusion
Python generators are a powerful feature that every developer should understand and utilize when appropriate. They offer significant advantages in terms of memory efficiency, performance, and code clarity. Whether you're processing large files, handling real-time data streams, or building complex pipelines, generators can help you write cleaner, more efficient Python code.
FAQs
Que 1. What is a Python generator, and how does it differ from a normal function?
Ans. A generator is a function that returns an iterator using the yield keyword. Unlike a normal function that returns a single value and exits, a generator can yield multiple values, one at a time, and resume execution between yields.
Que 2. How do Python generators improve memory efficiency?
Ans. Generators improve memory efficiency by generating values on the fly instead of storing the entire sequence in memory. This lazy evaluation ensures that only the current value is stored, making generators ideal for handling large datasets.
Que 3. Can you explain the concept of lazy evaluation in the context of generators?
Ans. Lazy evaluation refers to the technique of delaying the computation of a value until it is actually needed. Generators exemplify this by yielding values only when requested, avoiding unnecessary computations and memory usage.
Que 4. How can you convert a generator to a list?
Ans. You can convert a generator to a list using the list() function.
def pipeline_example(data):
for item in data:
yield item * 2
Que 5. What are some common use cases where generators are preferred over lists?
Ans. Generators are preferred in scenarios involving large datasets, infinite sequences, data streaming, and when building efficient data processing pipelines, as they avoid the memory overhead associated with lists.
About Author
Latest Blogs
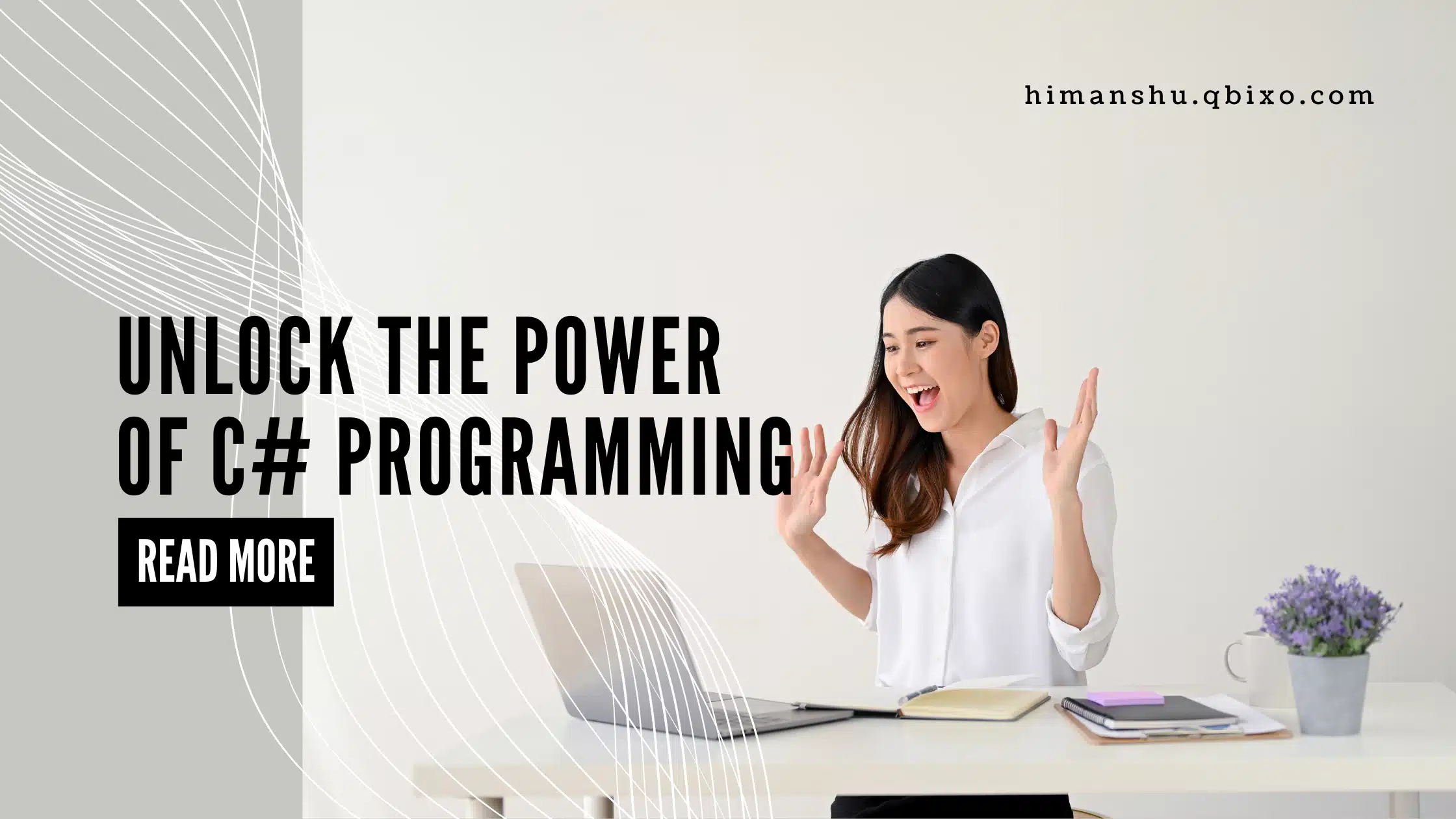
Mastering C#: Your Ultimate Guide to Learning C# Programming
Introduction to C#C# (pronounced "C sharp") is a versatile and powerful programming language developed by Microsoft. Launched in the early 2000s, it is primarily used for building Windows applications, web services, and games. With its clean syntax and object-oriented principles, C# has become one of the most popular programming languages worldwide.Why Learn C#?Versatility: C# is used in various domains, from desktop applications to cloud-based services.Strong Community: With a robust community …
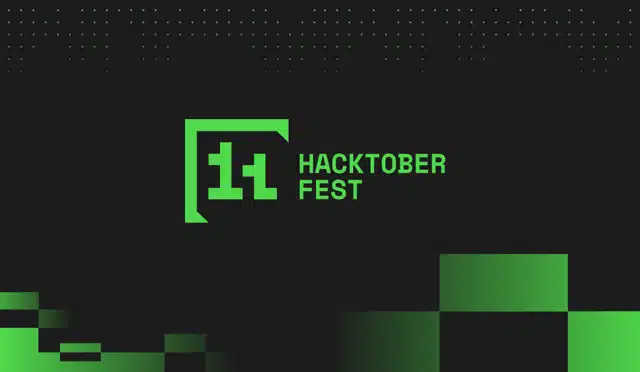
A Complete Guide to Hacktoberfest 2024: How to Register, Contribute, and Make the Most of It
Hacktoberfest is back for 2024! This annual event encourages developers worldwide to contribute to open-source projects. Whether you're a seasoned open-source contributor or a newcomer, this guide will walk you through the process of getting started, making contributions, and maximizing your participation in Hacktoberfest 2024. What is Hacktoberfest?Hacktoberfest is an event held every October to celebrate and promote open-source software. DigitalOcean organizes it in partnership with other tech companies and open-source …
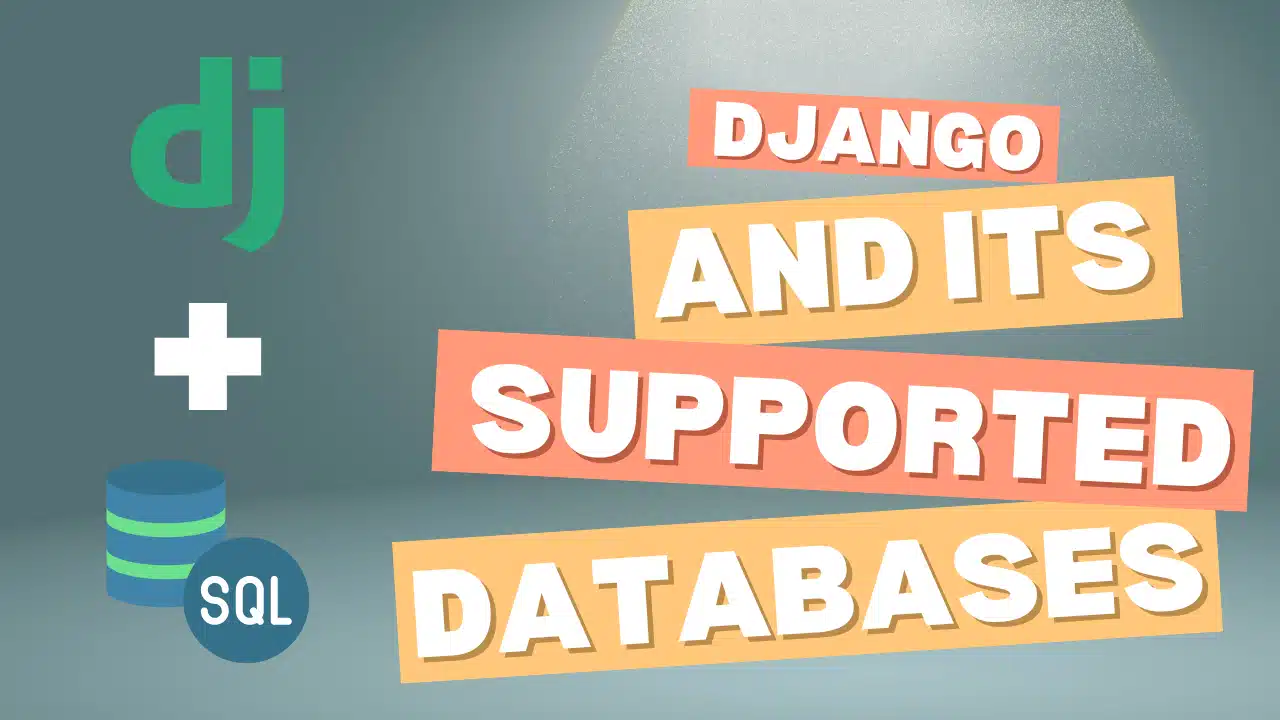
Django and Its Supported Databases: A Comprehensive Guide
Django, a powerful web framework written in Python, offers seamless integration with various databases. Choosing the right database depends on your project needs. This guide will explore all available databases compatible with Django, how to connect them, incompatible databases, and frequently asked interview questions related to Django database integration.Supported Databases in DjangoPostgreSQLMySQLMariaDBSQLiteOraclePostgreSQLPostgreSQL is a popular open-source relational database that is fully supported by Django. It's known for advanced features like …
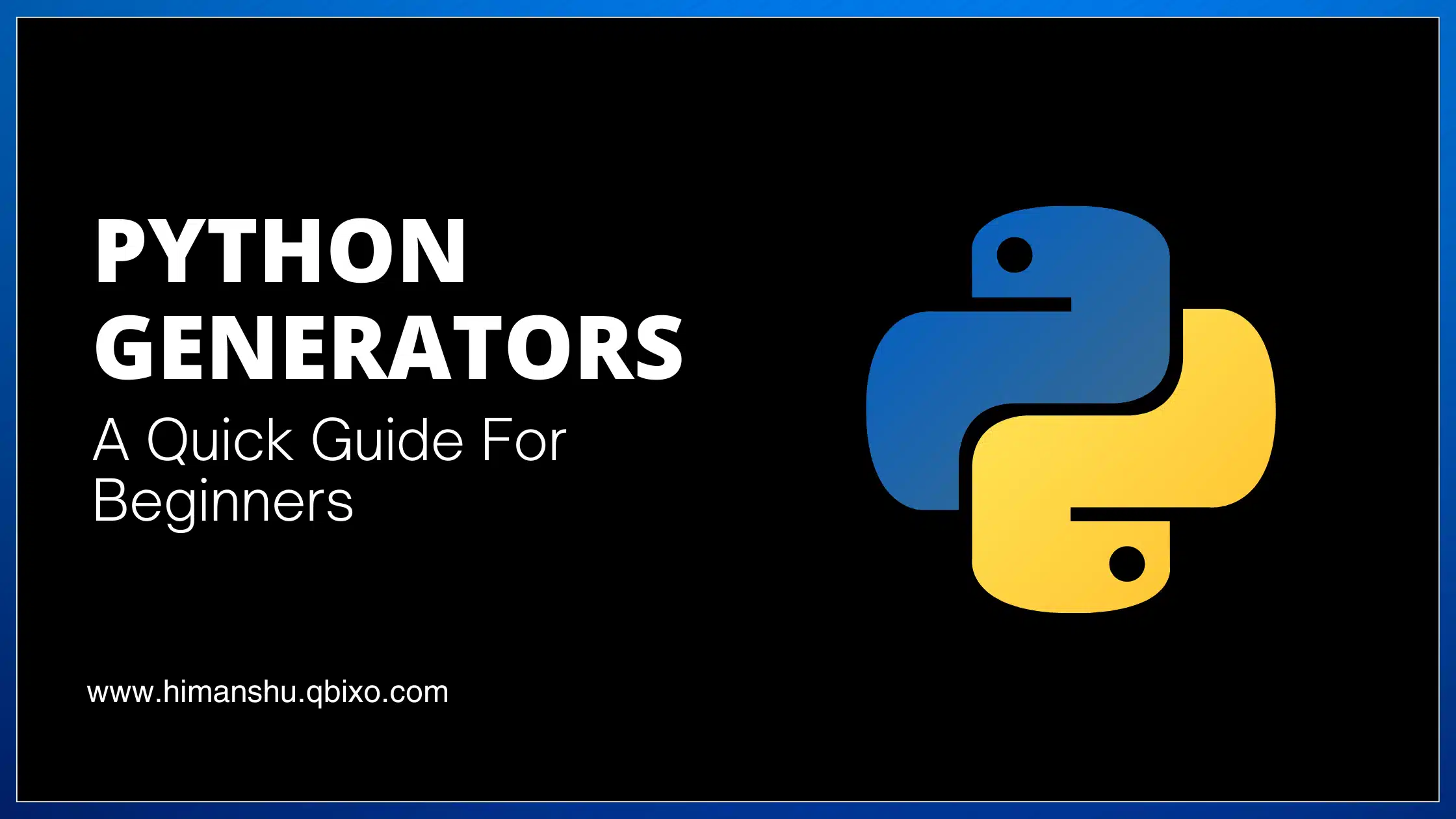
Python Generators: A Comprehensive Guide with Examples, Use Cases, and Interview Questions
IntroductionIn Python, generators provide a powerful tool for managing large datasets and enhancing performance through lazy evaluation. If you’re aiming to optimize memory usage or handle streams of data efficiently, understanding Python generators is crucial. This blog will cover what Python generators are, how they work, their advantages, scenarios where they shine, and some common interview questions. Whether you're a seasoned developer or new to Python, this guide will help …
Social Media
Tags
#Python Generators
#Python