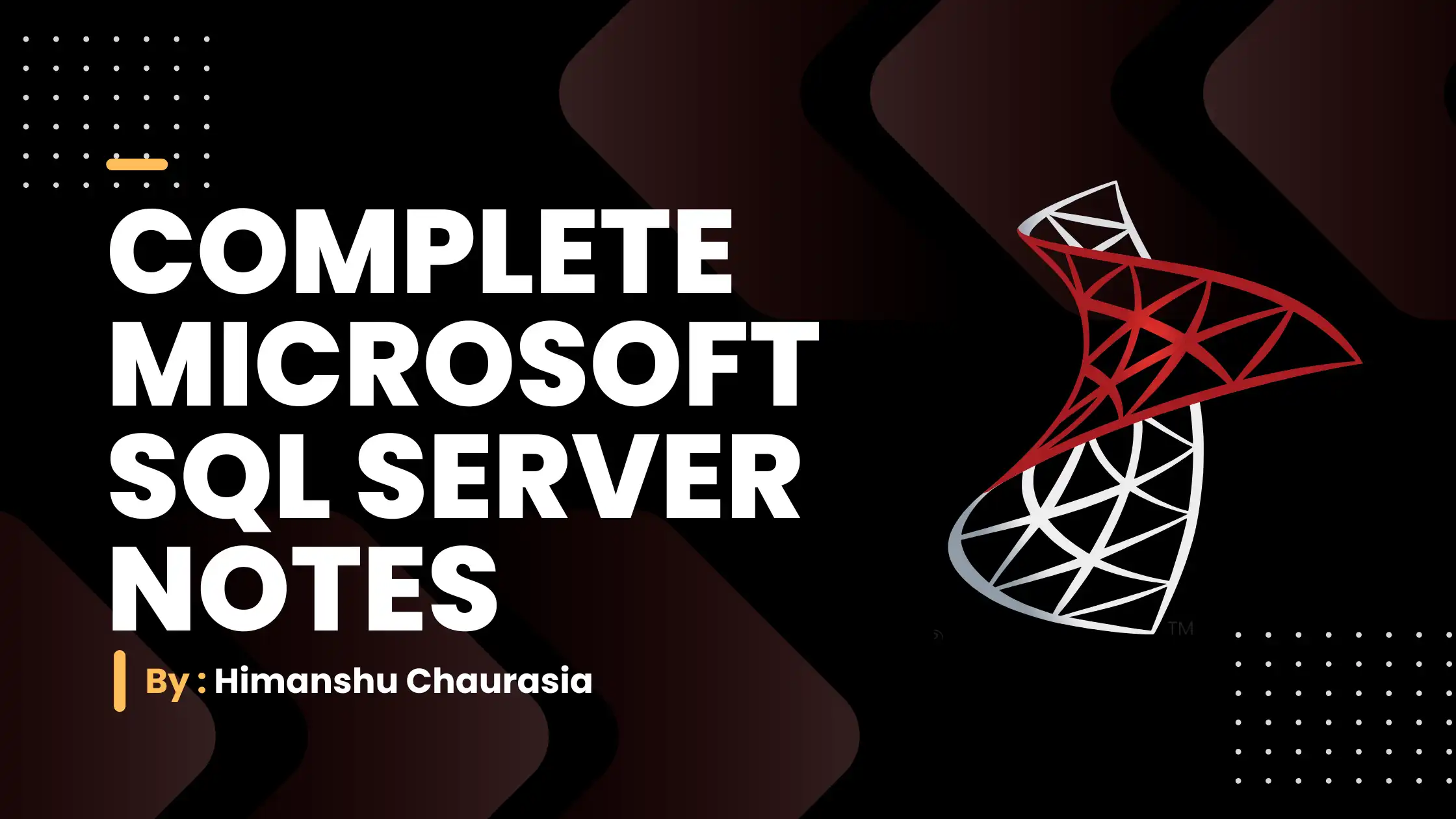
Complete Microsoft SQL Server Notes
1. Introduction to SQL Server
SQL Server is a relational database management system (RDBMS) developed by Microsoft. It is used for storing and retrieving data. SQL Server is known for its reliability, scalability, and powerful querying capabilities.
- SQL stands for Structured Query Language.
- SQL Server can be used for online transaction processing (OLTP), data warehousing, and more.
2. SQL Server Architecture
SQL Server follows a client-server architecture:
- Database Engine: The core service for storing, processing, and securing data.
- SQL Server Services: Handles security, backup, and access control.
- Query Processor: Responsible for interpreting SQL queries and generating execution plans.
3. Databases in SQL Server
A database in SQL Server is a container for tables, views, indexes, stored procedures, etc.
Creating a Database:
This creates a database named School.
4. Tables in SQL Server
A table is a collection of data organized into rows and columns. Each column represents a data type, and each row represents a record.
Creating a Table:
5. Data Types in SQL Server
SQL Server supports various data types:
- INT: Integer values.
- VARCHAR: Variable-length string.
- DATETIME: Date and time.
- FLOAT: Floating-point numbers.
- BIT: Boolean values (0 or 1).
Example:
6. Inserting Data into a Table
To insert data into a table, you use the INSERT INTO
statement.
Example:
7. Querying Data (SELECT)
The SELECT
statement is used to retrieve data.
Basic Query:
Select Specific Columns:
Filtering Data:
8. Updating Data (UPDATE)
To update existing data in a table, you use the UPDATE
statement.
Example:
9. Deleting Data (DELETE)
To delete data from a table, you use the DELETE
statement.
Example:
10. Joins in SQL Server
Joins combine rows from two or more tables based on a related column. SQL Server supports various types of joins:
1. INNER JOIN:
Returns rows when there is a match in both tables.
Example:
2. LEFT JOIN (LEFT OUTER JOIN):
Returns all records from the left table, and the matched records from the right table. If no match, NULL is returned for columns from the right table.
Example:
3. RIGHT JOIN (RIGHT OUTER JOIN):
Returns all records from the right table, and the matched records from the left table. If no match, NULL is returned for columns from the left table.
Example:
4. FULL OUTER JOIN:
Returns all records when there is a match in one of the tables.
Example:
5. CROSS JOIN:
Returns the Cartesian product of both tables (i.e., all possible combinations).
Example:
11. Aggregate Functions
SQL Server provides aggregate functions for summarizing data:
- COUNT(): Counts rows.
- SUM(): Sums numeric data.
- AVG(): Averages numeric data.
- MIN(): Returns the smallest value.
- MAX(): Returns the largest value.
Example:
12. Grouping Data (GROUP BY)
The GROUP BY
clause groups rows sharing the same value.
Example:
13. SQL Server Transactions
Transactions ensure that a set of operations are executed successfully as a single unit.
Begin Transaction:
If something goes wrong, you can use ROLLBACK
:
14. Views in SQL Server
A view is a virtual table, defined by a query.
Example:
You can query the view like a table
SELECT * FROM StudentDetails;
15. Indexes in SQL Server
Indexes improve the speed of data retrieval.
Example:
This query creates an index on the FirstName
column.
16. Stored Procedures
A Stored Procedure is a collection of SQL statements stored for later use.
Example:
You can call the stored procedure:
17. Triggers in SQL Server
A trigger is a special type of stored procedure that runs automatically when an event (INSERT, UPDATE, DELETE) occurs.
Example:
18. Normalization in SQL Server
Normalization reduces data redundancy and improves data integrity by organizing data into tables.
19. Backup and Restore in SQL Server
Backup and Restore are essential for data safety.
Backup Example:
Restore Example:
20. Basic Operations in SQL Server
Truncate Table:
TRUNCATE
removes all rows from a table but does not delete the table itself. It’s faster than DELETE
.
Drop Table:
DROP TABLE
deletes the table structure along with its data.
Drop Database:
DROP DATABASE
removes a database completely.
DROP DATABASE School;
Resetting Identity Column:
If a table has an IDENTITY
column, you can reset the identity value using DBCC CHECKIDENT
.
This resets the identity column of the Students
table to start from 1
again.
Frequently Asked Questions
Ans 1. SQL Server is a relational database management system (RDBMS) developed by Microsoft, known for its enterprise features, integration with Microsoft tools, and support for advanced analytics. MySQL is an open-source RDBMS, widely used for web applications, known for being lightweight and fast but with fewer advanced features than SQL Server.
Ans 2. A primary key is a field or a set of fields that uniquely identifies each row in a table. It ensures data integrity by preventing duplicate entries and helps in establishing relationships between tables.
Ans 3. The different types of joins in SQL Server are: 1. INNER JOIN: Returns rows when there is a match in both tables. 2. LEFT JOIN: Returns all rows from the left table and matching rows from the right table. 3. RIGHT JOIN: Returns all rows from the right table and matching rows from the left table. 4. FULL JOIN: Returns all rows when there is a match in one of the tables. 5. CROSS JOIN: Returns the Cartesian product of both tables.
Ans 4. The GROUP BY clause is used to group rows that have the same values into summary rows, such as finding the sum or average of a particular column. It’s often used with aggregate functions like COUNT, SUM, AVG, MAX, and MIN.
Ans 5. Normalization is the process of organizing data in a database to avoid redundancy and ensure data integrity. It involves dividing large tables into smaller tables and defining relationships between them. The goal is to reduce data anomalies and ensure efficient database design.