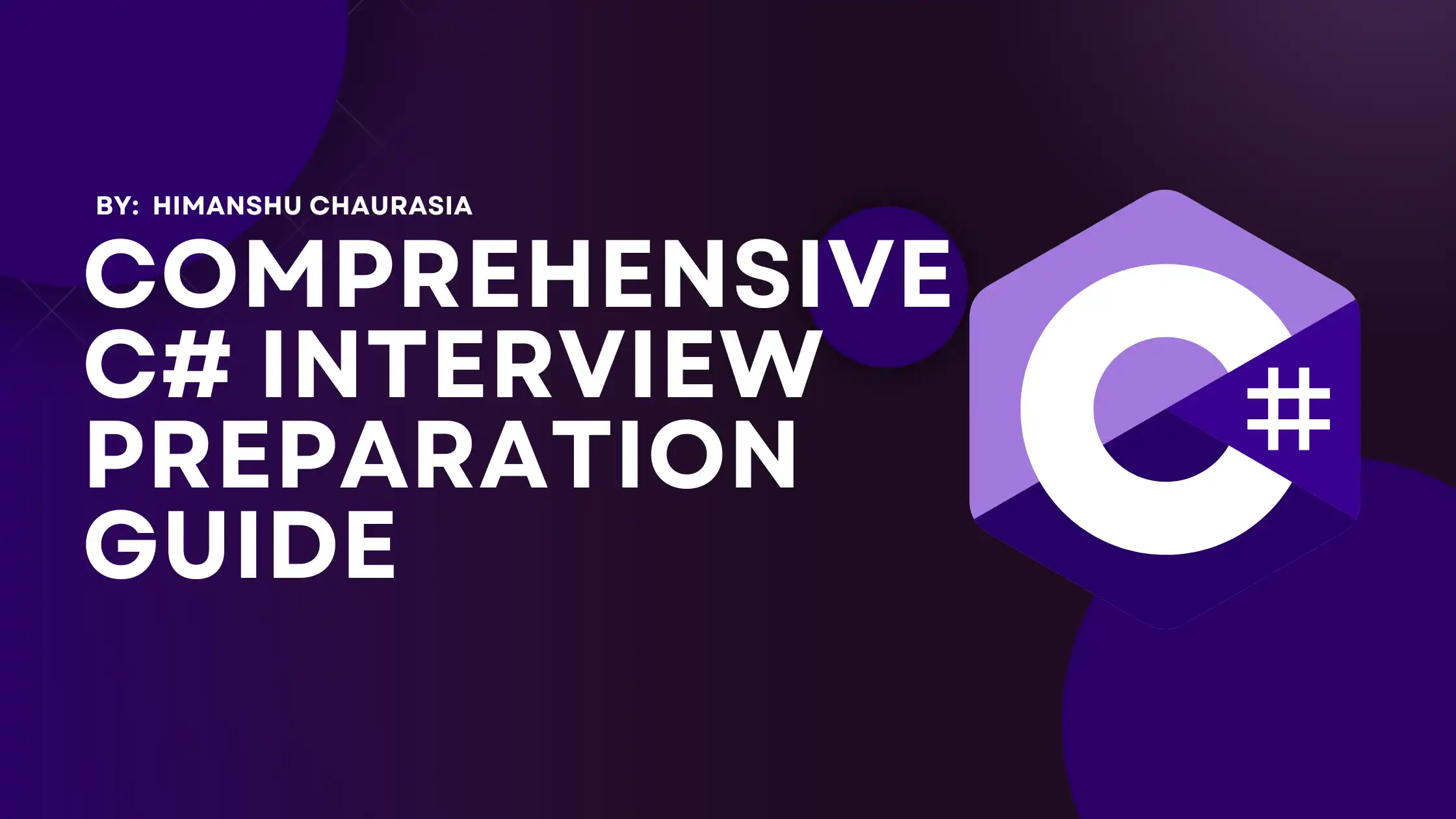
Comprehensive C# Interview Preparation Guide
1. What is C#?
C# (pronounced "C-Sharp") is a modern, object-oriented programming language developed by Microsoft. It is primarily used within the .NET framework for building robust, scalable applications that can run on multiple platforms, such as Windows, macOS, and Linux. C# supports key programming principles such as encapsulation, inheritance, polymorphism, and abstraction.
It can be used for a wide range of applications, from web and mobile to desktop and gaming. One of the most common architectural patterns used in C# applications is the MVC (Model-View-Controller) pattern, especially for web applications, where it helps in separating concerns and makes the application more maintainable.
2. What is a Data Type?
A data type in C# defines the type of data that can be stored in a variable. It also determines how much space is allocated in memory and how that data is processed. Data types can be classified into two categories: Primitive and Complex.
Primitive Data Types:
- int: Represents integer values (e.g., 5, -10).
- float: Represents decimal numbers with single precision (e.g., 3.14).
- char: A single Unicode character (e.g., 'A').
- string: Represents a sequence of characters (e.g., "Hello World").
- bool: Represents Boolean values, which can either be true or false.
Complex Data Types:
- object: Can store any type of data, as it is the root type of all other data types in C#.
- stringbuilder: A mutable sequence of characters that can be used for efficient string manipulation.
- enum: Represents a set of named constants (e.g.,
enum Days { Monday, Tuesday, Wednesday }
). - array: Represents a collection of variables of the same type.
- class: Defines properties and methods that together describe the behavior of an object.
- struct: A custom value type that groups related data together.
- DateTime: Represents date and time.
Memory Storage:
- Stack Memory: Used for value types like
int
,float
,bool
, etc. - Heap Memory: Used for reference types like
class
,string
, andobject
.
3. What is Boxing and Unboxing?
Boxing and Unboxing are operations that involve converting value types to reference types and vice versa.
Boxing: Converting a value type (e.g.,
int
) to a reference type (e.g.,object
).Example:
int num = 10; object obj = num; // Boxing
Unboxing: Converting a reference type back to a value type.
Example:
object obj = 10; int num = (int)obj; // Unboxing
4. What is a Literal?
A literal is a fixed value used directly in the code. It is a constant value assigned to variables. Literals are used to define constant data that doesn't change during the program's execution. Examples of literals are:
- Numeric literals:
5
,3.14
- String literals:
"Hello"
- Character literals:
'A'
5. What is an Operator?
An operator is a symbol used to perform operations on variables and values. Operators in C# can be divided into several types:
Unary Operators:
- Operate on a single operand.
- Examples:
++
,--
,!
,-
- Examples:
Binary Operators:
- Operate on two operands.
- Examples:
+
,-
,*
,/
,=
,==
- Examples:
Ternary Operator:
- Operates on three operands.
- Syntax:
condition ? value_if_true : value_if_false
- Syntax:
6. Conditional Statements
Conditional statements are used to make decisions based on conditions:
if-else: Executes different code based on conditions.
int number = 10; if (number > 0) Console.WriteLine("Positive"); else Console.WriteLine("Negative");
switch: Checks a variable against multiple values.
int day = 3; switch (day) { case 1: Console.WriteLine("Monday"); break; case 2: Console.WriteLine("Tuesday"); break; default: Console.WriteLine("Unknown Day"); }
7. Loops
A loop allows you to repeatedly execute a block of code. C# provides several types of loops:
for loop: Ideal when you know how many times to loop.
for (int i = 1; i <= 5; i++) Console.WriteLine("Hello World");
while loop: Used when the number of repetitions is unknown.
int i = 1; while (i <= 5) { Console.WriteLine("Hello World"); i++; }
do-while loop: Executes the loop at least once before checking the condition.
int i = 1; do { Console.WriteLine("Hello World"); i++; } while (i <= 5);
foreach loop: Iterates over a collection like arrays or lists.
int[] numbers = { 1, 2, 3, 4, 5 }; foreach (int num in numbers) Console.WriteLine(num);
8. Arrays
Arrays are used to store multiple values in a single variable. In C#, there are several types of arrays:
One-Dimensional Array: Stores values in a linear structure.
int[] numbers = new int[5]; numbers[0] = 10;
Two-Dimensional Array: A matrix-like structure, stores values in rows and columns.
int[,] matrix = new int[2, 3]; matrix[0, 0] = 1;
Jagged Array: An array of arrays where each inner array can have different sizes.
int[][] jaggedArray = new int[3][]; jaggedArray[0] = new int[] { 1, 2 };
Object Array: Stores elements of different types.
object[] objArray = new object[3]; objArray[0] = 10; objArray[1] = "Hello";
9. Object-Oriented Programming (OOP) Concepts
Object-oriented programming (OOP) is a paradigm that uses objects and classes to structure the code.
Core OOP Concepts:
Inheritance: A class can inherit properties and methods from another class.
class Animal { public void Eat() { } } class Dog : Animal { public void Bark() { } }
Encapsulation: Hides the internal state of an object and only exposes a controlled interface.
class Person { private int age; public void SetAge(int value) { if (value >= 0) age = value; } public int GetAge() => age; }
Polymorphism: Allows methods to have different implementations based on the object calling them.
Method Overloading:
public int Add(int a, int b) => a + b; public double Add(double a, double b) => a + b;
Method Overriding:
class Animal { public virtual void Sound() { } } class Dog : Animal { public override void Sound() => Console.WriteLine("Bark"); }
Abstraction: Hides complex implementation details and exposes only necessary features.
abstract class Shape { public abstract void Draw(); } class Circle : Shape { public override void Draw() => Console.WriteLine("Drawing Circle"); }
10. Access Modifiers
Access modifiers control the visibility of class members. The most commonly used modifiers are:
- Public: The member is accessible from anywhere.
- Private: The member is accessible only within the class.
- Protected: The member is accessible within the class and derived classes.
11. Types of Classes in C#
C# supports several types of classes:
- Concrete Class: A class that can be instantiated.
- Abstract Class: A class that cannot be instantiated directly and is designed to be inherited.
- Static Class: A class that cannot be instantiated and contains only static members.
12. Constructor
A constructor is a special method used to initialize objects. Constructors have the same name as the class and can be parameterless or parameterized.
class Person
{
public string Name;
public int Age;
public Person(string name, int age)
{
Name = name;
Age = age;
}
}
13. Interfaces vs Abstract Classes
- Abstract Classes: Can have implemented methods and are meant to be inherited by other classes. A class can inherit only one abstract class.
- Interfaces: Cannot have method implementations and are used to define a contract that classes must adhere to. A class can implement multiple interfaces.
14. Exception Handling
Exception handling helps manage runtime errors to prevent the application from crashing.
- try-catch-finally blocks:
try
: Code that may throw an exception.catch
: Code to handle the exception.finally
: Code that will always execute, regardless of whether an exception occurred.
try { int result = 10 / 0; }
catch (DivideByZeroException ex) { Console.WriteLine("Cannot divide by zero!"); }
finally { Console.WriteLine("Execution finished."); }
15. Collections in C#
A collection is an object that holds multiple objects. C# provides several types:
- List<T>: A generic collection for storing elements of a specific type.
- Dictionary<TKey, TValue>: Stores key-value pairs.
- Queue<T>: A FIFO (first-in, first-out) collection.
Stack<T>: A LIFO (last-in, first-out) collection.
List<int> numbers = new List<int> { 1, 2, 3, 4 };
Additional C# Concepts (Not Covered Yet):
Delegates:
A delegate is a type that represents references to methods with a specific parameter list and return type. It allows methods to be passed as parameters.
delegate void PrintMessage(string message); PrintMessage print = (message) => Console.WriteLine(message); print("Hello, C#");
Events:
An event is a mechanism to provide notifications in response to an action or change. It is built on top of delegates and is primarily used in GUI applications (like Windows Forms or WPF).
class Publisher { public event EventHandler DataProcessed; public void ProcessData() { // Processing logic here DataProcessed?.Invoke(this, EventArgs.Empty); } }
LINQ (Language Integrated Query):
LINQ is a set of methods that allows querying collections in C#. It enables writing SQL-like queries directly in C# code to filter, sort, and manipulate data.
int[] numbers = { 1, 2, 3, 4, 5 }; var evenNumbers = from num in numbers where num % 2 == 0 select num; foreach (var num in evenNumbers) Console.WriteLine(num);
Async and Await (Asynchronous Programming):
The
async
andawait
keywords are used for handling asynchronous operations, which allows for non-blocking I/O operations (e.g., file handling, web requests).public async Task<string> GetDataFromAPI() { HttpClient client = new HttpClient(); string data = await client.GetStringAsync("https://api.example.com"); return data; }
Reflection:
Reflection allows inspecting and interacting with object types and metadata at runtime. You can get the type of an object, invoke methods, or access properties dynamically.
Type type = typeof(MyClass); PropertyInfo prop = type.GetProperty("MyProperty"); Console.WriteLine(prop.GetValue(myObject));
Dependency Injection (DI):
- Dependency Injection is a design pattern used to manage class dependencies by providing them externally rather than creating them inside the class.
DI is commonly used in ASP.NET Core for better testability and loose coupling.
public class MyService { private readonly IMyDependency _myDependency; public MyService(IMyDependency myDependency) => _myDependency = myDependency; }
Nullable Types:
C# allows value types to be nullable, meaning they can hold a
null
value (useful when working with databases or APIs wherenull
might be a valid value).int? nullableInt = null;
Garbage Collection (GC):
Garbage Collection in C# is the process of automatically reclaiming memory that is no longer in use by the program. This is a key feature of .NET for memory management.
GC.Collect(); // Force garbage collection (rarely necessary)
Tuple Types:
A Tuple is a data structure that can hold multiple values of different types without creating a custom class.
var tuple = Tuple.Create(1, "hello", true); Console.WriteLine(tuple.Item1); // Outputs: 1
String Interpolation:
String interpolation allows embedding expressions inside string literals for more readable and concise string formatting.
int age = 30; string message = $"I am {age} years old.";
Frequently Asked Questions
Ans 1.
In C#, there are two main categories of data types: value types and reference types.
-
Value types store the actual value directly in memory. Examples include
int
,char
,struct
, andbool
. When you assign one value type to another, it copies the actual value.Example:
int a = 5; int b = a; // b gets a copy of the value of a
-
Reference types store a reference to the memory location where the data is stored. Examples include
class
,string
,object
, andarray
. When you assign one reference type to another, both variables point to the same memory location.Example:
class Person { public string Name; } Person p1 = new Person { Name = "John" }; Person p2 = p1; // p2 references the same object as p1
Ans 2.
Abstract Class: An abstract class can have both fully implemented methods and abstract methods (methods without a body). A class can only inherit one abstract class, and it can provide a common base implementation for other derived classes.
-
Example:
abstract class Animal { public void Eat() { Console.WriteLine("Eating..."); } public abstract void MakeSound(); // Abstract method }
-
Interface: An interface only contains method signatures and properties, without implementation. A class can implement multiple interfaces, and it must provide its own implementation for all the methods defined in the interface.
Example:
interface IAnimal { void MakeSound(); }
The key difference is that a class can implement multiple interfaces but can only inherit one abstract class. Additionally, interfaces cannot have implemented methods, whereas abstract classes can.
Ans 3.
The using
keyword in C# has two main uses:
-
Importing namespaces: The
using
keyword allows you to use types from a specified namespace without needing to write the fully qualified name.Example:
using System; // No need to write System.Console.WriteLine Console.WriteLine("Hello World!");
-
Ensuring proper resource disposal (using statements): The
using
statement is used to define a scope for objects that implementIDisposable
(e.g., file streams, database connections). It ensures that resources are automatically cleaned up when the block is exited.Example:
using (StreamReader reader = new StreamReader("file.txt")) { string line = reader.ReadLine(); Console.WriteLine(line); } // reader.Dispose() is automatically called here.
Ans 4.
In C#, the ==
operator and the Equals()
method are used to compare objects, but they work differently:
-
==
Operator: Compares two operands for equality. For primitive types, it compares values directly. For reference types (like objects), it compares the memory addresses (references) unless the equality operator is overloaded in a class.Example:
string str1 = "Hello"; string str2 = "Hello"; bool result = (str1 == str2); // true, because both strings have the same value
-
Equals()
Method: Compares the contents or values of two objects. For reference types, it checks if the objects' values are equal, and for value types, it compares the actual values.Example:
string str1 = "Hello"; string str2 = new string("Hello".ToCharArray()); bool result = str1.Equals(str2); // true, because both strings have the same value
In general, Equals()
is preferred for comparing the actual contents of objects, while ==
is used to check if two references point to the same memory location or if the operator is overloaded.