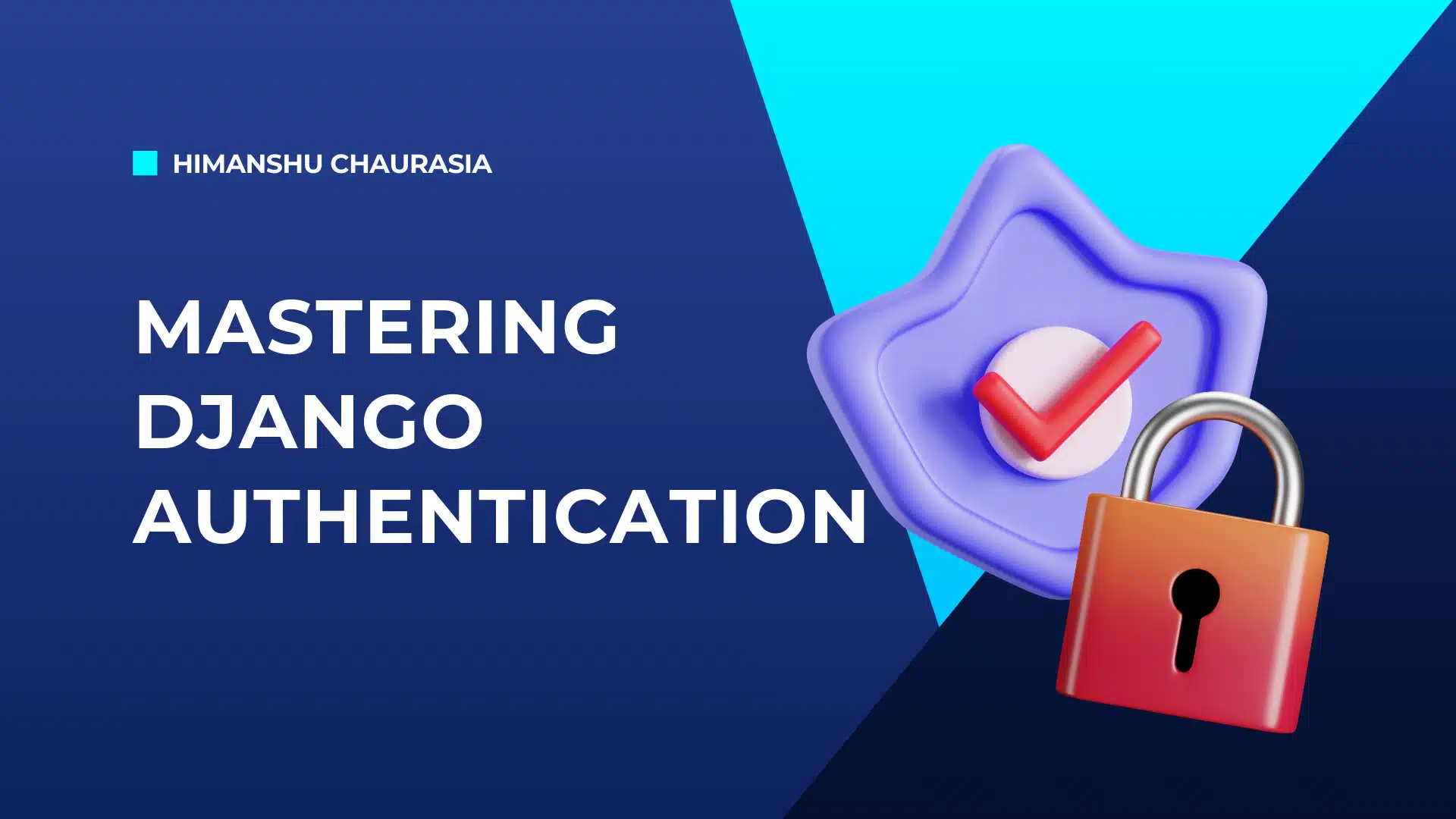
Mastering Django Authentication: Comprehensive Guide with Code Examples
Introduction
Django is a high-level Python web framework known for its simplicity and powerful features. One of its core functionalities is a robust authentication system. This guide aims to provide a comprehensive understanding of Django authentication, with detailed explanations and code examples. Whether you're a beginner or an experienced developer, this tutorial will help you become proficient in Django authentication."
Table of Contents
|
1. Introduction to Django Authentication
Django’s authentication system handles user authentication and authorization, providing built-in views and forms for login, logout, and password management. It includes:
- User management (registration, login, logout)
- Password management (reset, change)
- Authentication backends for custom authentication methods
2. Setting Up Django Project
First, ensure you have Django installed. If not, you can install it using pip:
pip install django
Create a new Django project and app:
django-admin startproject myproject
cd myproject
django-admin startapp accounts
Add the accounts
app to your INSTALLED_APPS
in settings.py
:
# myproject/settings.py
INSTALLED_APPS = [
...
'accounts',
...
]
3. Django Authentication System Overview
Django’s authentication system is built around the User
model, which includes fields like username
, password
, email
, etc. Django also provides authentication views and forms.
4. User Registration
Create a registration form in accounts/forms.py
:
# accounts/forms.py
from django import forms
from django.contrib.auth.models import User
class UserRegistrationForm(forms.ModelForm):
password = forms.CharField(label='Password', widget=forms.PasswordInput)
password2 = forms.CharField(label='Repeat password', widget=forms.PasswordInput)
class Meta:
model = User
fields = ('username', 'first_name', 'email')
def clean_password2(self):
cd = self.cleaned_data
if cd['password'] != cd['password2']:
raise forms.ValidationError('Passwords don’t match.')
return cd['password2']
Create a registration view in accounts/views.py
:
# accounts/views.py
from django.shortcuts import render, redirect
from .forms import UserRegistrationForm
def register(request):
if request.method == 'POST':
form = UserRegistrationForm(request.POST)
if form.is_valid():
new_user = form.save(commit=False)
new_user.set_password(form.cleaned_data['password'])
new_user.save()
return redirect('login')
else:
form = UserRegistrationForm()
return render(request, 'accounts/register.html', {'form': form})
Add the registration URL in accounts/urls.py
:
# accounts/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('register/', views.register, name='register'),
]
Update the project's urls.py
to include accounts
URLs:
# myproject/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accounts.urls')),
]
Create a registration template in accounts/templates/accounts/register.html
:
<!-- accounts/templates/accounts/register.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Register</h2>
<form method="post">
{{ form.as_p }}
{% csrf_token %}
<button type="submit">Register</button>
</form>
{% endblock %}
5. User Login and Logout
Django provides built-in views for login and logout. Update accounts/urls.py
to include these views:
# accounts/urls.py
from django.urls import path
from . import views
from django.contrib.auth import views as auth_views
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
]
Create a login template in accounts/templates/registration/login.html
:
<!-- accounts/templates/registration/login.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Login</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Login</button>
</form>
{% endblock %}
Create a logout template in accounts/templates/registration/logged_out.html
:
<!-- accounts/templates/registration/logged_out.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>You have been logged out</h2>
<a href="{% url 'login' %}">Login again</a>
{% endblock %}
6. Password Management
Django includes built-in views for password reset and change. Update accounts/urls.py
:
# accounts/urls.py
from django.urls import path
from . import views
from django.contrib.auth import views as auth_views
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
path('password_reset/', auth_views.PasswordResetView.as_view(), name='password_reset'),
path('password_reset/done/', auth_views.PasswordResetDoneView.as_view(), name='password_reset_done'),
path('reset/<uidb64>/<token>/', auth_views.PasswordResetConfirmView.as_view(), name='password_reset_confirm'),
path('reset/done/', auth_views.PasswordResetCompleteView.as_view(), name='password_reset_complete'),
path('password_change/', auth_views.PasswordChangeView.as_view(), name='password_change'),
path('password_change/done/', auth_views.PasswordChangeDoneView.as_view(), name='password_change_done'),
]
Create templates for password reset and change views. Example for password reset:
<!-- accounts/templates/registration/password_reset_form.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Reset your password</h2>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Reset password</button>
</form>
{% endblock %}
7. User Profile Management
Create a view to handle user profiles:
# accounts/views.py
from django.contrib.auth.decorators import login_required
@login_required
def profile(request):
return render(request, 'accounts/profile.html')
Add a URL for the profile view in accounts/urls.py
:
# accounts/urls.py
urlpatterns = [
path('register/', views.register, name='register'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
path('profile/', views.profile, name='profile'),
path('password_reset/', auth_views.PasswordResetView.as_view(), name='password_reset'),
path('password_reset/done/', auth_views.PasswordResetDoneView.as_view(), name='password_reset_done'),
path('reset/<uidb64>/<token>/', auth_views.PasswordResetConfirmView.as_view(), name='password_reset_confirm'),
path('reset/done/', auth_views.PasswordResetCompleteView.as_view(), name='password_reset_complete'),
path('password_change/', auth_views.PasswordChangeView.as_view(), name='password_change'),
path('password_change/done/', auth_views.PasswordChangeDoneView.as_view(), name='password_change_done'),
]
Create a profile template in accounts/templates/accounts/profile.html
:
<!-- accounts/templates/accounts/profile.html -->
{% extends "base_generic.html" %}
{% block content %}
<h2>Profile</h2>
<p>Welcome, {{ user.username }}!</p>
{% endblock %}
8. Custom Authentication Backends
Django allows the creation of custom authentication backends. Create a custom backend in accounts/backends.py
:
# accounts/backends.py
from django.contrib.auth.backends import BaseBackend
from django.contrib.auth.models import User
class EmailBackend(BaseBackend):
def authenticate(self, request, username=None, password=None, **kwargs):
try:
user = User.objects.get(email=username)
if user.check_password(password):
return user
except User.DoesNotExist:
return None
def get_user(self, user_id):
try:
return User.objects.get(pk=user_id)
except User.DoesNotExist:
return None
Add the custom backend to AUTHENTICATION_BACKENDS
in settings.py
:
# myproject/settings.py
AUTHENTICATION_BACKENDS = [
'accounts.backends.EmailBackend',
'django.contrib.auth.backends.ModelBackend',
]
Django's authentication system is powerful and flexible, providing essential features for secure user management. By following this guide, you now have a comprehensive understanding of how to implement and customize Django authentication.
Mastering Django authentication is crucial for building secure and user-friendly applications. Keep exploring and enhancing your skills to create robust Django applications.
Frequently Asked Questions
Ans 1. Use Django’s AbstractUser or AbstractBaseUser to create a custom user model.
Ans 2. Use third-party packages like django-allauth or social-auth-app-django
Ans 3. Use Django’s built-in email system to send verification emails and create views to handle the verification process.