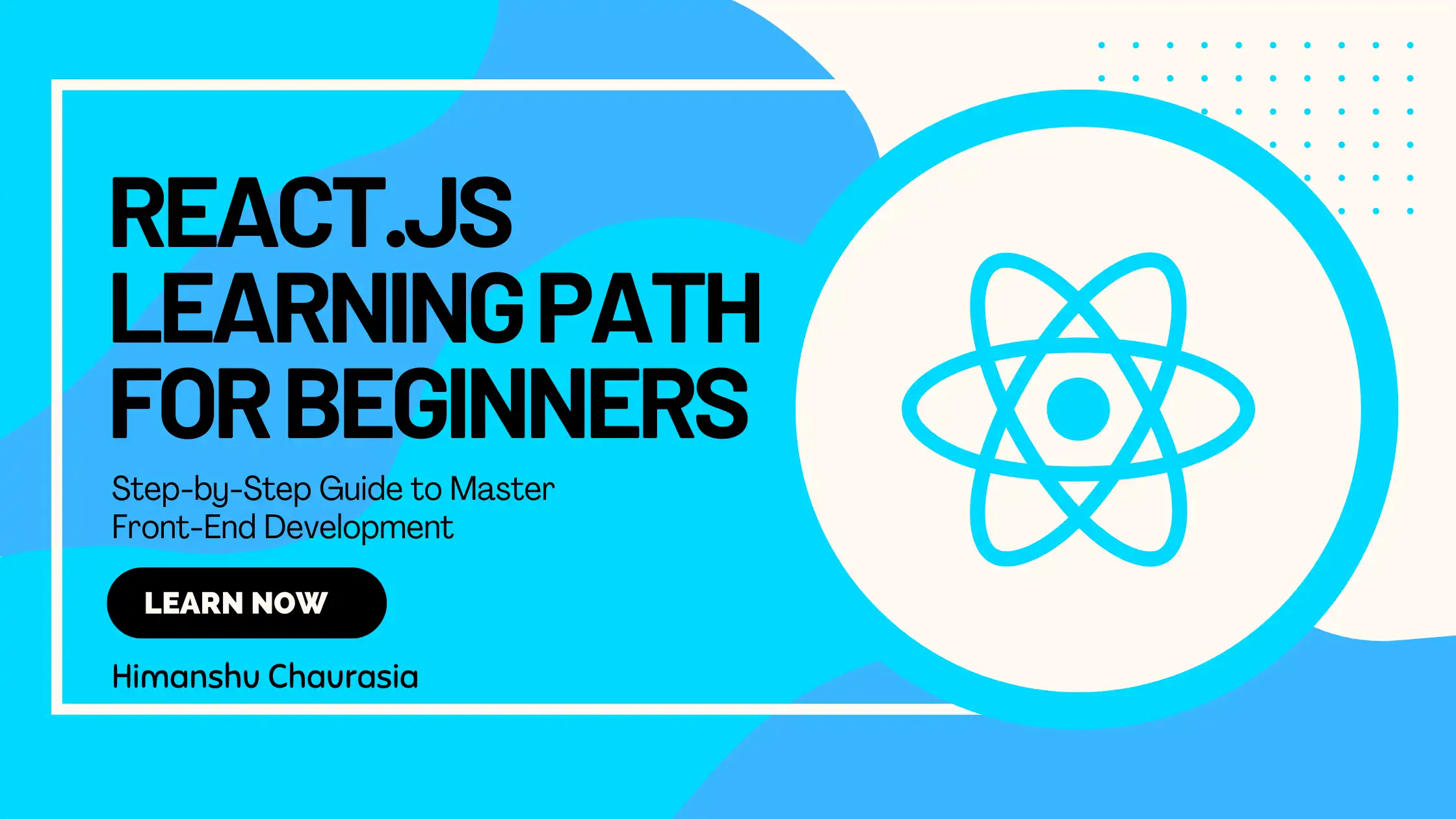
🚀 React.js Learning Path for Beginners: Step-by-Step Guide to Front-End Mastery
React.js is one of the most popular JavaScript libraries used to build modern, fast, and dynamic web applications. If you're a beginner and wondering where to start, this simple and structured guide will help you learn React step-by-step.
1. 🧠 What is React.js?
React.js is an open-source JavaScript library developed by Facebook. It’s used to build interactive UIs for web and mobile apps using reusable components.
2. 🎯 Why Learn React.js?
📈 High demand in web development jobs
🧩 Component-based architecture
⚡ Fast performance using Virtual DOM
🌍 Supported by a large community
📱 Can build mobile apps using React Native
3. 🧰 Basic Requirements Before Learning React
Before starting React, you should know:
✅ HTML – Structure of web pages
✅ CSS – Styling and layout
✅ JavaScript (ES6+) – Variables, functions, arrays, objects, arrow functions, and
fetch
4. 🛣️ React.js Learning Path (Step-by-Step)
📌 Step 1: Learn the Basics of JavaScript (ES6+)
Understand:
let
,const
Arrow functions
Destructuring
Promises and
fetch()
📌 Step 2: Understand How React Works
What is JSX
Difference between Functional & Class Components
Props & State
Conditional Rendering
Handling Events
📌 Step 3: Set Up Your React Project
Use Create React App:
Or use Vite for faster setup.
📌 Step 4: Learn React Hooks
Hooks allow functional components to manage state and lifecycle:
useState
useEffect
useRef
useContext
📌 Step 5: Add Navigation with React Router
Use:
<BrowserRouter>
<Route>
<Link>
📌 Step 6: Connect to APIs
Learn to use fetch()
or axios
inside useEffect()
to get dynamic data.
📌 Step 7: Styling in React
Choose one:
CSS Modules
Styled Components
Tailwind CSS
Sass
📌 Step 8: Organize Code and Components
Create reusable components
Maintain a clear folder structure
Use Context API for state management
📌 Step 9: Build Projects
Hands-on practice is key. Start with:
To-do App
Weather App
Notes App
Portfolio Website
📌 Step 10: Deploy Your App
Use:
Netlify
Vercel
GitHub Pages
5. 🎁 Bonus Tips
Learn TypeScript with React for better type safety
Use VS Code with React-specific extensions
Follow the official React documentation
Join communities like Stack Overflow, Reddit, and Twitter
Frequently Asked Questions
Ans 1. Yes, React is beginner-friendly, especially if you know JavaScript basics.
Ans 2. With consistent practice, you can learn React basics in 4–6 weeks.
Ans 3. No, start with the Context API; learn Redux once you're comfortable with React basics.
Ans 4. Yes! You can use React Native to build mobile apps using the same knowledge.